If you are a company with hundreds or thousands of workstations or you have a lot of remote branches, then we are sure that your maintenance tasks are huge and time demanding. Especially when we are talking about installation of desktop terminal client, implementing various additional elements, custom configurations etc.
With Green Screens Web Terminal maintenance is minimal, almost non-existing.
Already we got a lot of questions how to do this or how to do that for automation tasks. The most common question is how to simplify remote configuration especially when there are custom security requirements.
Let us describe couple of scenarios:
The simplest approach
Send group email to Green Screens Web Terminal main login url where workstation operators must enter UUID, HOST and optional parameters like DISPLAY NAME, user login data etc. like shown on the image below. Here workstation operator need to enter some login parameters.
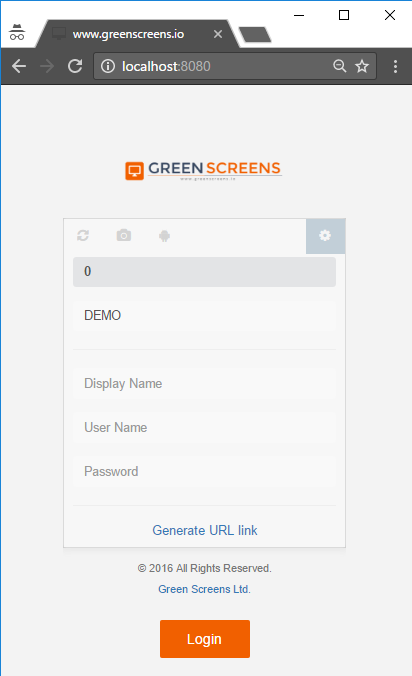
When connected, workstation operator can bookmark generated URL link and later open web terminal directly without entering any information.
Encrypted web terminal URL with UUID and HOST looks like shown on image below.
This approach generally works quite well, but with one small limitation. Because of security reasons, username and password can not be bookmarked. If encrypted terminal URL contains username and password, they are time limited to few seconds and reuse limited. (can be used only once).
But what if we don't want workstation operator to know about login parameters at all?
Better approach
Well, to answer that question is very simple. Use some scripting to generate web terminal access URLs. Even better, each user can have their own URL link with specific parameters like DISPLAY NAME, PROGRAM, MENU, LIB etc. With this, access can be controlled in telnet exit programs so that workstation operators can't share links which increase security and enable terminal session control.
Of course, that kind of feature significantly reduce administration tasks. All you need to do is to generate links and send them to workstation operators email. For example, generate CSV document with username, email and display names. Load CSV document into PHP, read line by line and generate URL connection link with user specific data and send it to users email.
First, let's create csv file
0,DEMO,QSECOFR,DSPQSEC01;DSPQSEC02,admin@greenscreens.io
0,DEMO,JOHN,DSPJHN01;DSPJHN02,john@greenscreens.io
Then some simple PHP csv reader
<?php
/**
* Copyright (C) 2015, 2016 Green Screens Ltd.
*
* PHP lib demo to create Green Screens Web Terminal encrypted URL
*
*/
require_once "funcs.php";
// Green Screens RSA service URL to get encryption public key
$GREENSCREENS_SERVICE = "http://localhost:8080";
$CSV = "./sample.csv";
/*
* Read CSV file and process line by line
*/
function doCSV($fileName)
{
$csvFile = file($fileName);
$data = [];
foreach ($csvFile as $line) {
$data[] = str_getcsv($line);
$pieces = explode(";", $data[0][3]);
foreach ($pieces as $piece) {
encryptCSVLine($data[0][0], $data[0][1], $data[0][2], $piece);
// get email from $data[0][4] and send it to the user
}
}
}
/*
* Encrypt CSV line into URL
*/
function encryptCSVLine($uuid, $host, $user, $displayName)
{
global $GREENSCREENS_SERVICE;
global $UUID;
global $HOST;
$json_data = array('uuid' => $uuid,
'host' => $host,
'user' => $user,
'displayName' => $displayName
);
$data = json_encode($json_data);
$json = encryptJson($GREENSCREENS_SERVICE, $data);
// generate http://localhost:9080/lite?d=[HEX encrypted AES]&k=[RSA encrypted AES]
$url = jsonToURLService($GREENSCREENS_SERVICE, $json);
print $url;
print "\n";
return $url;
}
doCSV($CSV)
?>
For you we have prepared simple working example written in PHP with Green Screens PHP Encryption API. You can find it on our GitHub account here PHP Encrypter