Yes you read it right. It is very possible. Green Screens as a web terminal can easily show images. For example, product images based on product code from your ERP application.
There are various ways to do it and it can divided it in two groups:
ways to show an image
- popup screen
- embedded in screen
- open in new tab
ways to detect image selector
- double click and RPG
- mouse region detection and screen parsing
Ways to show an image
Green Screens Terminal is web based so standard HTML IMG tag and/or custom CSS styling are all what is needed. Only question is which of them we will use and how.
Popup screen
Use Tn5250.Prompt.message or Tn5250.Prompt.notify API by generating IMG html element with url to image.
For example:
Tn5250.message('SKU:12345', '<img src="http://server/images/12345.png" />')
This code can be also executed from 5250 STRRMTCMD like this:
STRPCCMD CMD('script:Tn5250.message(''SKU:12345'', ''<img src="http://server/images/12345.png" />'')')
Embedded in screen
Embedded image means adding custom css style to html element. In this case to .tngrid which is main html element where 5250 screen is generated.
$('.tngrid').css({
'background-image': 'url(http://www.greenscreens.io/blog/images/logo.png) !important',
'background-repeat': 'no-repeat !important',
'background-position': '20px 95% !important',
'background-size': '150px !important'
});
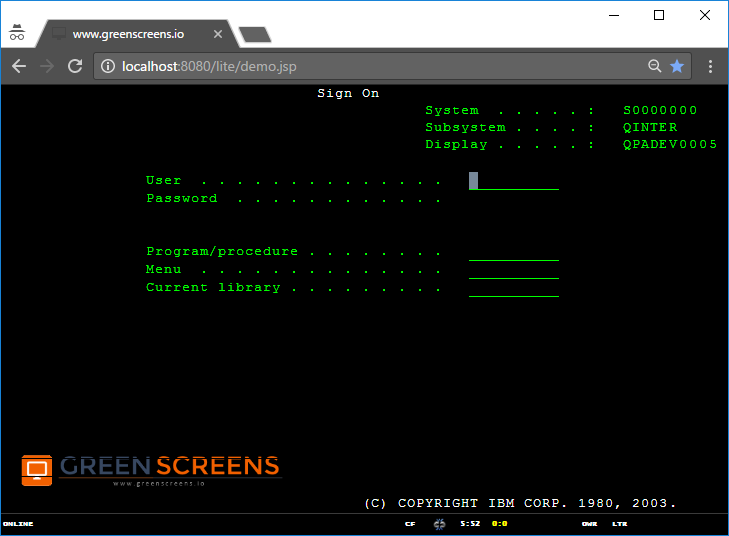
Example from above can be wrapped inside custom function like:
function showImage(url) {
$('.tngrid').css({
'background-image': 'url(' + url + ') !important',
'background-repeat': 'no-repeat !important',
'background-position': '20px 50px !important',
'background-size': '300px !important'
});
}
And then use it from STRRMTCMD
STRPCCMD CMD('script:showImage("http://server/images/12345.png"')
Open in new tab
Use remote command to open url link in new tab.
STRPCCMD CMD("http://server/images/12345.png")
Ways to detect image code
Ways to detect image code / product code are in a fact detection and extraction of the text on the screen.
Double click and RPG
Well, it does not have to be RPG, it can be any other program at host side. Crucial is double click! Mouse double click on screen area will send ROW/COL position to remote host and that data can be used inside RPG (or any other program) to get cursor position request. Base on that data program knows on which record inside subfile workstation operator clicked meaning we know for which record operator want's to show the data.
Now, only what is needed is to call  STRRMTCMD as shown in previous paragraphs to popup image or to open it in another browser tab.
Mouse region detection and screen parsing
For screen parsing there are 2 options available
// Parse binary screen data into JSON and get text array obj.text
var screen = Tn5250.Application.getScreen();
var obj = Tn5250.Parser.screenToJson(screen);
// get text from specific range
var text = Tn5250.Parser.textRangeExtract(screen, 4, 6, 12, 6);
All we need here is mouse move detection on document and focused row/col detection based on mouse position. If row/col is in specific range, pickup text from that range by previous example. Use data to generate image URL.
var cfg = Tn5250.Application.getConfig();
$(document).on ('mousemove', function(e){
// detect cfg.attrHash, cfg.fldHash for valid screen
// if hash is from screen with product codes continue
var t = Tn5250.GUI.findEl(e.clientX, e.clientY, 'TD', cfg);
if (t) {
var col = t.cellIndex;
var row = t.parentNode.rowIndex;
// send remote command for row col position so that RPG
// can detect cursor postioning and start remote command to show image
Tn5250.Application.sendCursor(e, cfg, row, col);
}
});